일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 항해99
- java
- sw expert academy
- redux-toolkit
- C++
- 코딩테스트합격자되기
- 알고리즘
- 리액트
- 자바
- react-router
- 이코테
- 항해플러스
- Get
- 테코테코
- JavaScript
- 매일메일
- json-server
- maeil-mail
- Python
- redux-saga
- axios
- 프로그래머스
- useDispatch
- SW
- react
- redux
- programmers
- createSlice
- Algorithm
- react-redux
- Today
- Total
목록React/게시판만들기 v1. (21)
Binary Journey
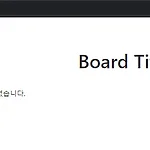
목차 돌아가기: binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial [React][CRUD] create-board-tutorial code: github.com/jwlee-lnd/react-create-board jwlee-lnd/react-create-board Description(korean) : https://binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial - jwlee-lnd/react-create-boar.. binaryjourney.tistory.com board 목록 조회를 데이터 존재 유무 상관없이 가능하도록 해놨기 때문에 서버를 실행시키지 않았거나 board..
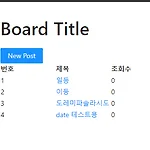
목차 돌아가기: binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial [React][CRUD] create-board-tutorial code: github.com/jwlee-lnd/react-create-board jwlee-lnd/react-create-board Description(korean) : https://binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial - jwlee-lnd/react-create-boar.. binaryjourney.tistory.com 9편에서는 게시글 한 개의 내용을 불러오는 것을 다뤘고 이번 편에서는 게시글 목록 전체 조회를 다룰 것이다. 이..
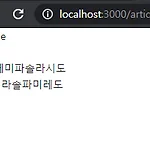
목차 돌아가기: binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial [React][CRUD] create-board-tutorial code: github.com/jwlee-lnd/react-create-board jwlee-lnd/react-create-board Description(korean) : https://binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial - jwlee-lnd/react-create-boar.. binaryjourney.tistory.com 화면 이동이 해결되었으니 이제 내가 등록한 글의 내용이 화면에 나타나는 것을 구현해보도록 해보자 첫번째로 art..
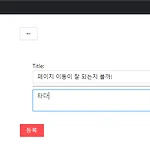
목차 돌아가기: binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial [React][CRUD] create-board-tutorial code: github.com/jwlee-lnd/react-create-board jwlee-lnd/react-create-board Description(korean) : https://binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial - jwlee-lnd/react-create-boar.. binaryjourney.tistory.com saga 로 이용 중인 파일에서는 react-router-dom이 바로 import 되지 않는 것으로 알고 있다..
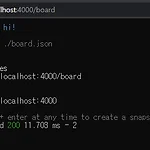
목차 돌아가기: binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial [React][CRUD] create-board-tutorial code: github.com/jwlee-lnd/react-create-board jwlee-lnd/react-create-board Description(korean) : https://binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial - jwlee-lnd/react-create-boar.. binaryjourney.tistory.com 들어가기 전에 이전까지 만든 소스에 문제가 없는지 (블로그 포스팅하면서 내가 소스 수정하고 빼먹은 부분이 있을 ..
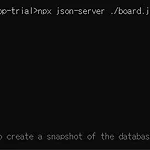
목차 돌아가기: binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial [React][CRUD] create-board-tutorial code: github.com/jwlee-lnd/react-create-board jwlee-lnd/react-create-board Description(korean) : https://binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial - jwlee-lnd/react-create-boar.. binaryjourney.tistory.com 게시글 저장 구현에 본격적으로 들어가기 전에 임시 서버를 띄워보도록 하겠다. 그나마 과정이 간단한 json-se..
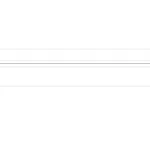
목차 돌아가기: binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial [React][CRUD] create-board-tutorial code: github.com/jwlee-lnd/react-create-board jwlee-lnd/react-create-board Description(korean) : https://binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial - jwlee-lnd/react-create-boar.. binaryjourney.tistory.com 시간이 부족한 관계로 서버 연동 + 저장 기능은 가능하면 저녁 이후 혹은 내일에 해야 할 것 같다. 남은 시간 ..
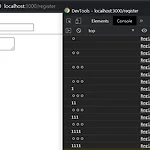
목차 돌아가기: binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial [React][CRUD] create-board-tutorial code: github.com/jwlee-lnd/react-create-board jwlee-lnd/react-create-board Description(korean) : https://binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial - jwlee-lnd/react-create-boar.. binaryjourney.tistory.com 3편에서 RegisterPage.js 를 글 수정할 때도 재사용할 것이라고 언급한 적이 있었는데 Register..
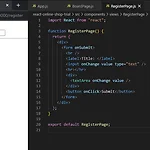
목차 돌아가기: binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial [React][CRUD] create-board-tutorial code: github.com/jwlee-lnd/react-create-board jwlee-lnd/react-create-board Description(korean) : https://binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial - jwlee-lnd/react-create-boar.. binaryjourney.tistory.com * (11/6) useState보다 useSelector 사용을 더 추천합니다. 이번에는 게시글 저장을 구현해보..
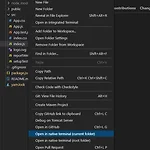
목차 돌아가기: binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial [React][CRUD] create-board-tutorial code: github.com/jwlee-lnd/react-create-board jwlee-lnd/react-create-board Description(korean) : https://binaryjourney.tistory.com/pages/ReactCRUD-create-board-tutorial - jwlee-lnd/react-create-boar.. binaryjourney.tistory.com VSCode extension 중 open native terminal 을 설치하면 root folder 와 현..